Array Collections
So far the arrays we used were simple lists of elements of a single type. This is sufficient for many situations, but there are problems that they can’t solve.
If you need to know the order of distances of points to a given position y a simpel array will not help. It can be sorted, but you will lose the index information and don’t know which actual point is the closest or farthest.
A solution for this can be found in Array Collections. These are containers that hold any number of arrays of the same length. Array Collections can help with a lot of problems that include multiple elements with a complex data structure.
Let’s say you want to store positions and you also want to store information alongside them, like a color, weight or even a name. A classic solution would be something like a struct or tuple, where you create a single data structure that holds all this information and then make an array from it. While this works it is also extremely inefficient when it comes to any kind of operation you want to do on the list.
The alternative is to store all these properties in own arrays and then bundle the arrays in an own structure for easier handling. This is what Array Collections do and it is what is basically used within Geometry data, though those are a bit more complex.
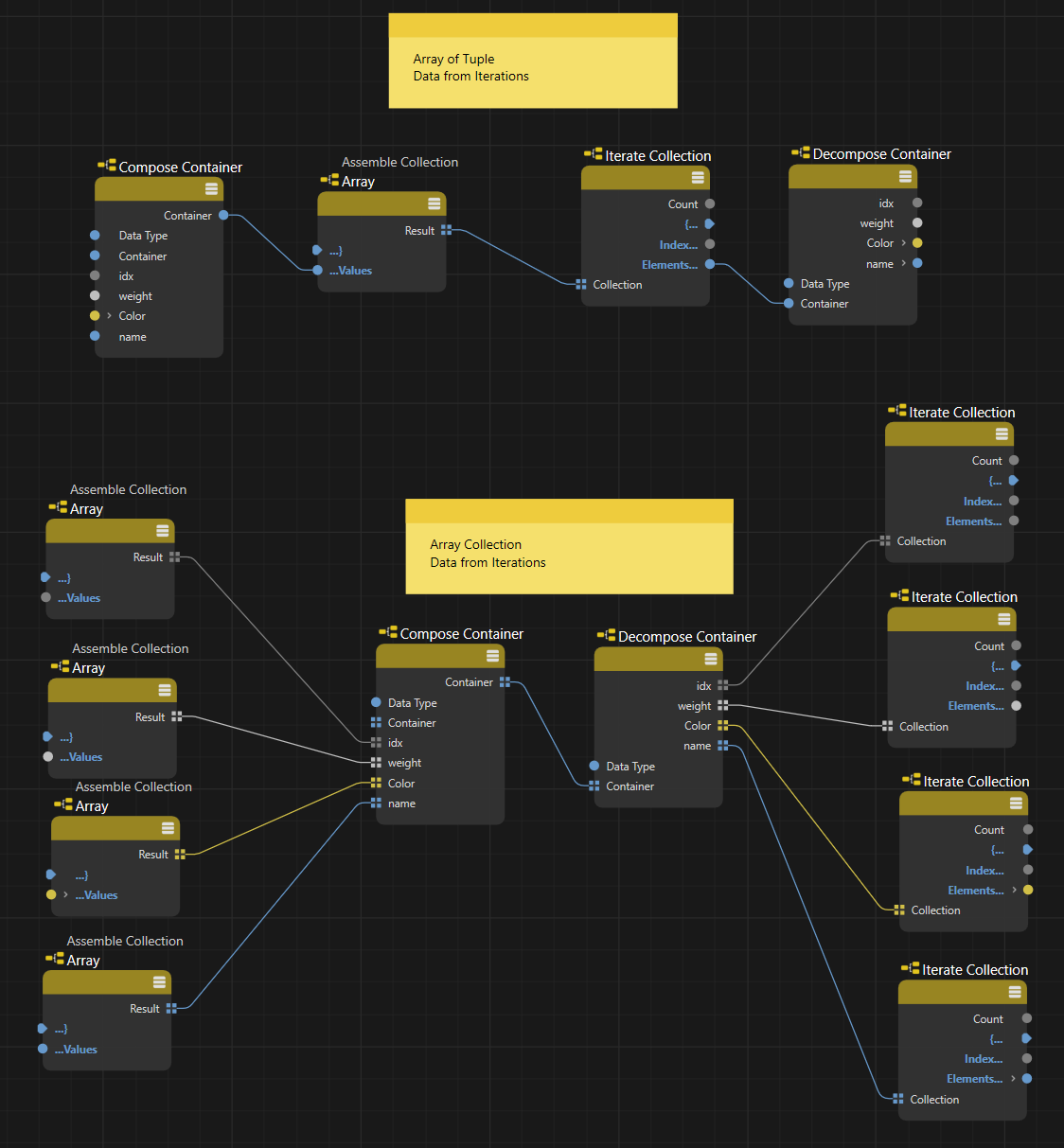
On first look the Array Collection seems more complicated, but that only depends on if you already work with Arrays or not. If you already get most your data as arrays and later continue to process it as array this can look quite different.
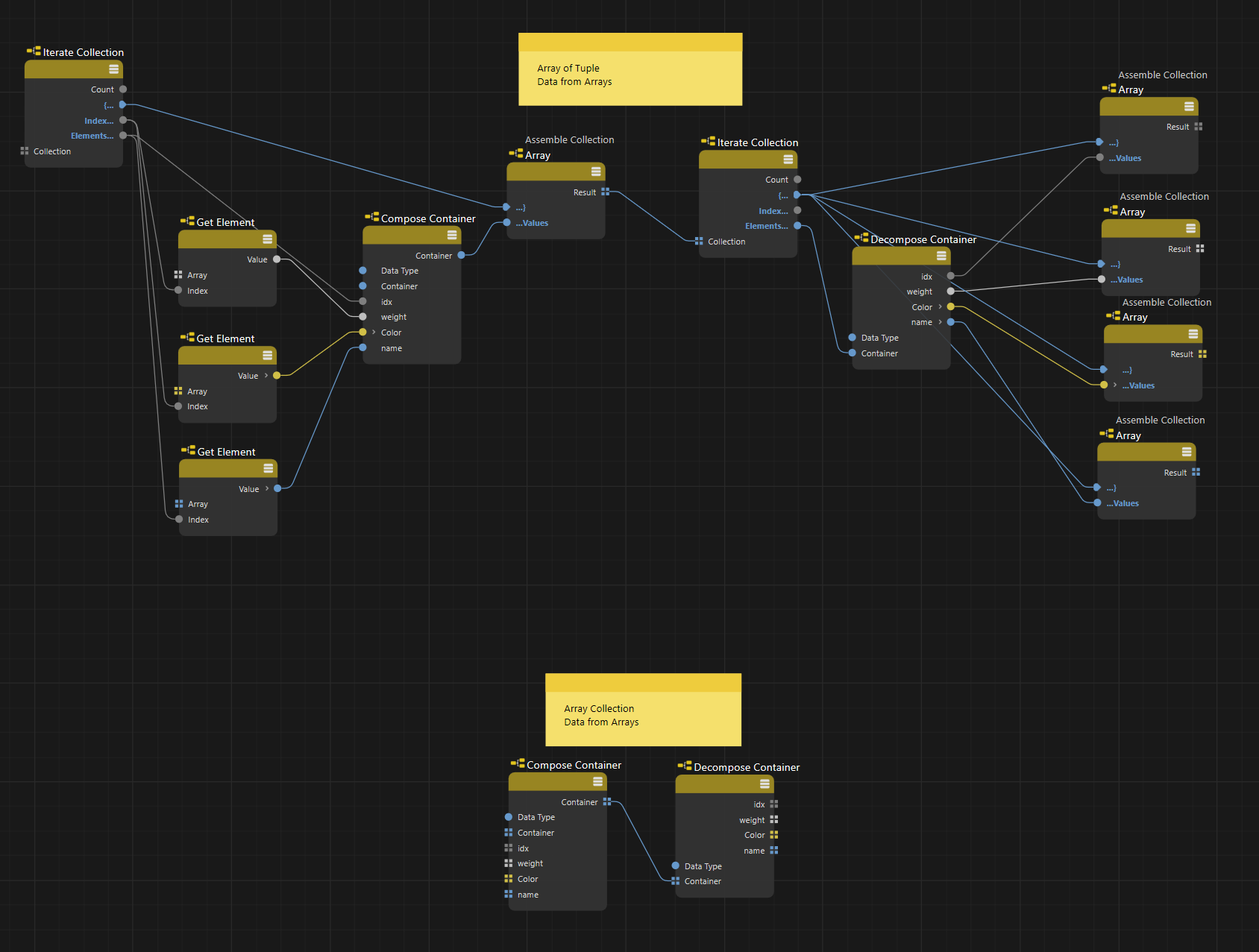
If you don’t plan to do many operations on the array of tuples, like changing individual elements weights or similar, using arrays of tuples can reduce the number of connections you need between function blocks, if however you either already have the data in single arrays or you do need to modify individual values using array collections makes things faster and easier.
The basic array functions Get Element and Set Element work on array collections just like on arrays. The only difference is that you get more than one data port.
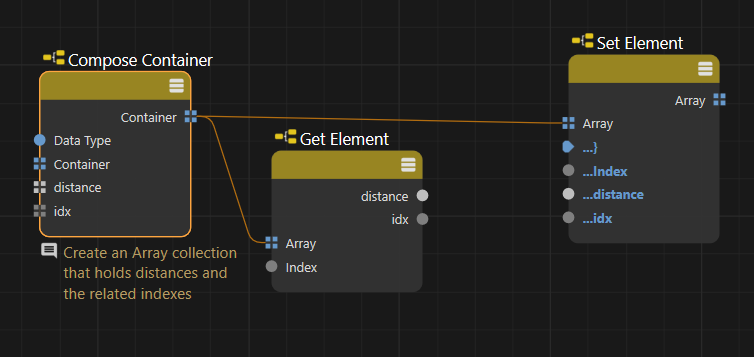
Example-Comparison-Tuples-Array-Collections.c4d (not a working scene, just the base of the screenshots for your entertainment)
Using array collections for sorting
Coming back to the original problem of sorting, this is how Array Collections can help solving it.
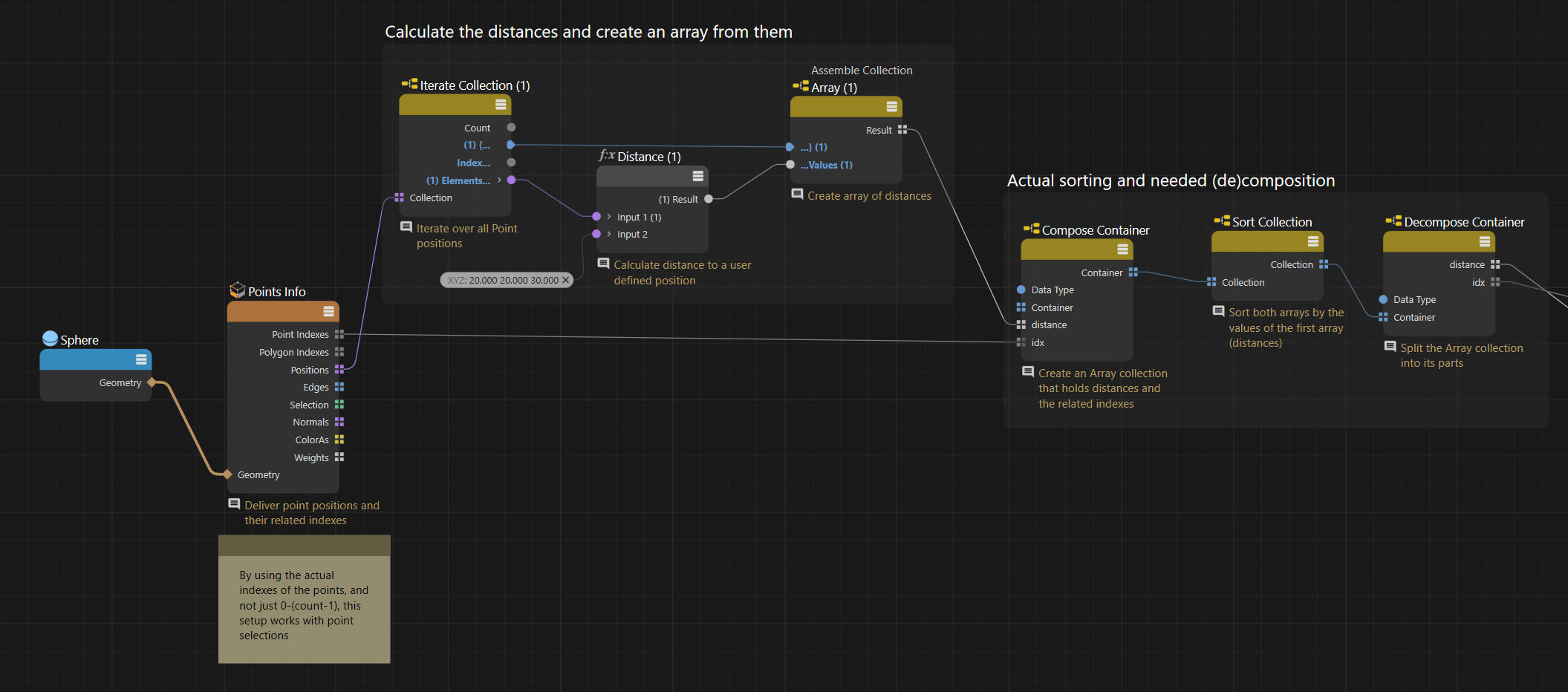
Only the three nodes on the right are what we actually need to sort the two arrays of indexes and distances, the left part is only there to provide the test data.
Compose Container takes the two arrays and encapsulates them, Sort Collection then sorts the collection. Which internal array should be the base of the sorting is indicated either by it’s number in order (index) or by name. If the set name is not part of the array the sorting will fail so it often makes sense to use the index to prevent later problems from renaming.
If you want to work with CSV data within Scene Nodes you will have to work with Array Collections, because this is what the Import Data node delivers.