Inserting Elements into an Array
The Insert Element node can insert any number of identical values at a given index into an array. While this sounds straight forward enough there are some pitfalls, mainly you have to observe that the length of the array changes with each insertion. This is especially important if you iterate over this array and while doing so insert elements depending on the data read from the array, this will very likely go wrong.
A simple trick to avoid this problem is to iterate over the array backwards, this way only the indexes of already processed elements change.
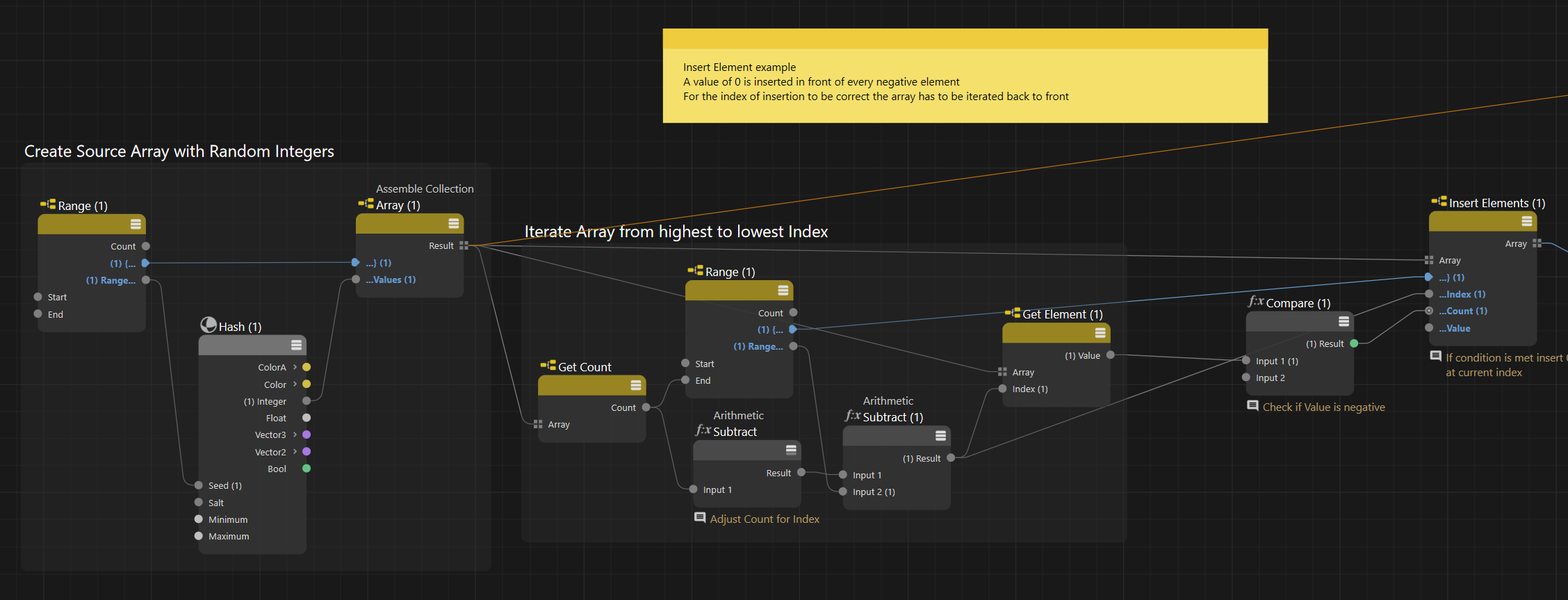
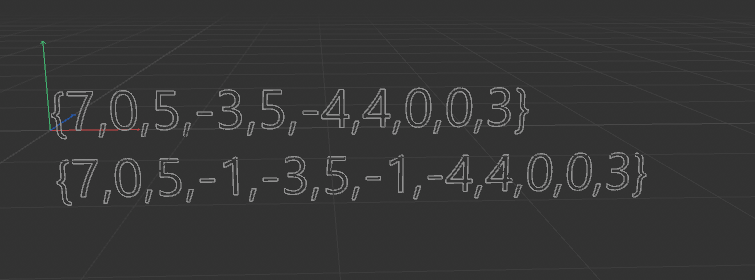
In the attached example every occurrence of a negative value in the array will result in a new element with the value 0 being inserted in front of it.
Example-Insert-Element-into-Array.c4d
Deleting Elements from an Array
Erase Element allows for the removal of any number of array elements at a given index. The example here shows a conditional removal of elements. If the value of an element is below 0 it is removed.
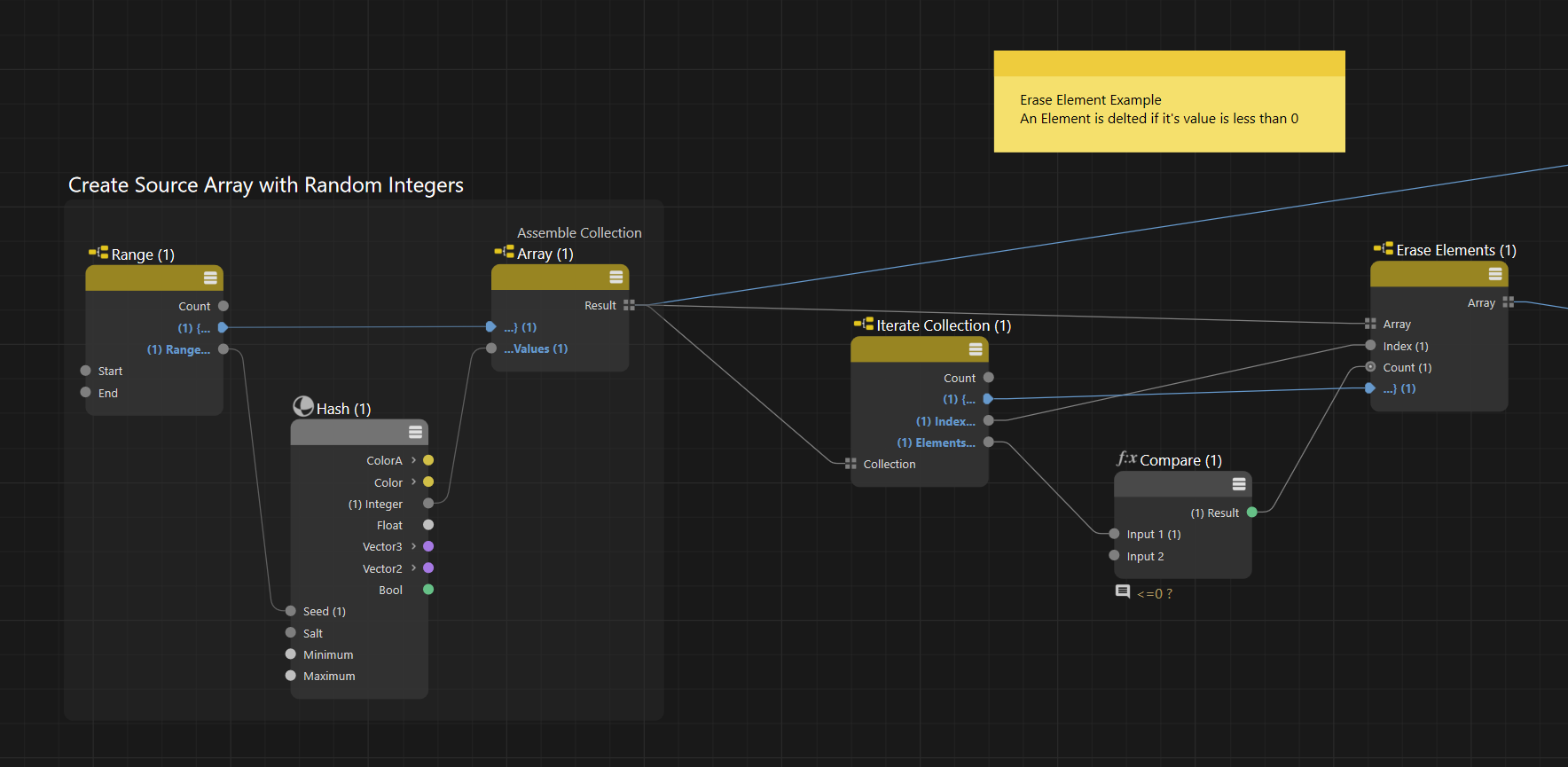
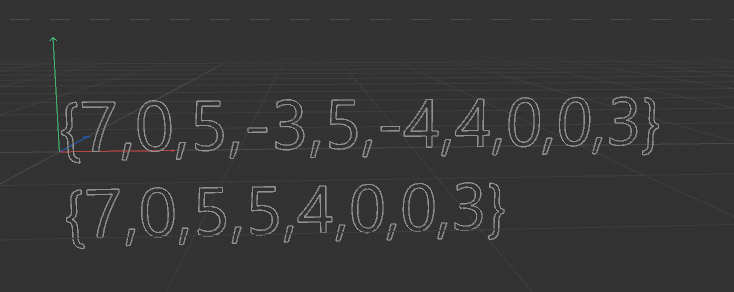
Try the Keep Order option in the Erase Elements node. It is on by default and ensures that the order of remaining elements is the same as before. This might sound curious, but due to automatic parallelization of the execution it would otherwise happen that the output order changes. In cases where the order of values is of no importance this is an option for speed optimization.
Example-Erase-Element-from-Array.c4d
Swap Erase Elements
This is a rather specific node, it allows to replace an element at a given index by the last value(s) of the array. This is mostly for performance reasons and can be used in situations where the order of elements is unimportant. Removing the last elements of an array is much faster than removing them from somewhere within the array, to the point that swapping the values and then deleting the last element is a lot faster than a simple erase.
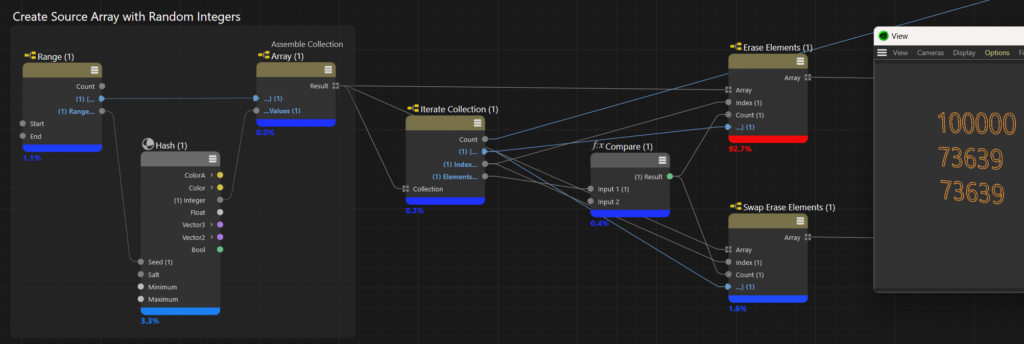
With sufficiently high array lengths the difference in speed is extreme, in case of 100.000 elements the speed factor between the two this example is 50.
The big downside of Swap Erase is that it does not retain the order of elements.
Truncating an Array
While it is possible to remove any number of elements from an Array using Erase Element this can be rather slow. For a performant reduction of an Array by cutting off from the end of it the Truncate node is preferable.
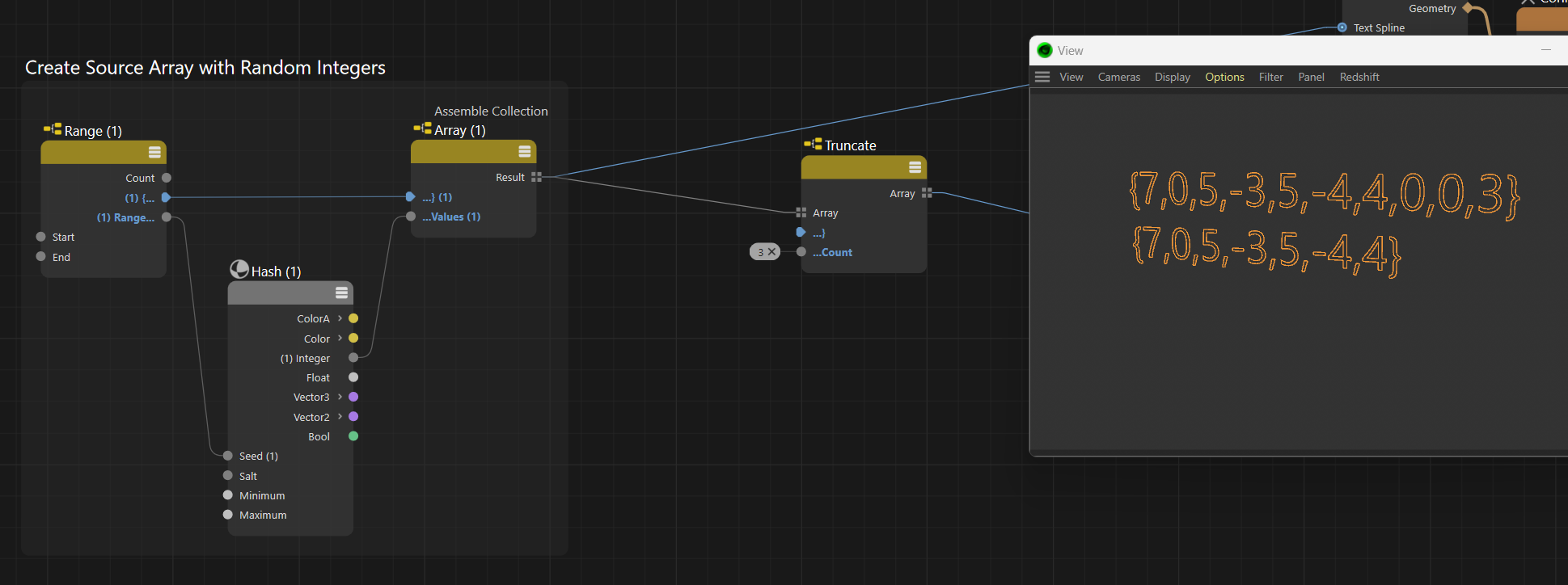
Changing the Order of Arrays
By default there are three ways to change the order of an array, first by simply reversing the order and second by sorting it by values and last not least a random shuffle of the elements.
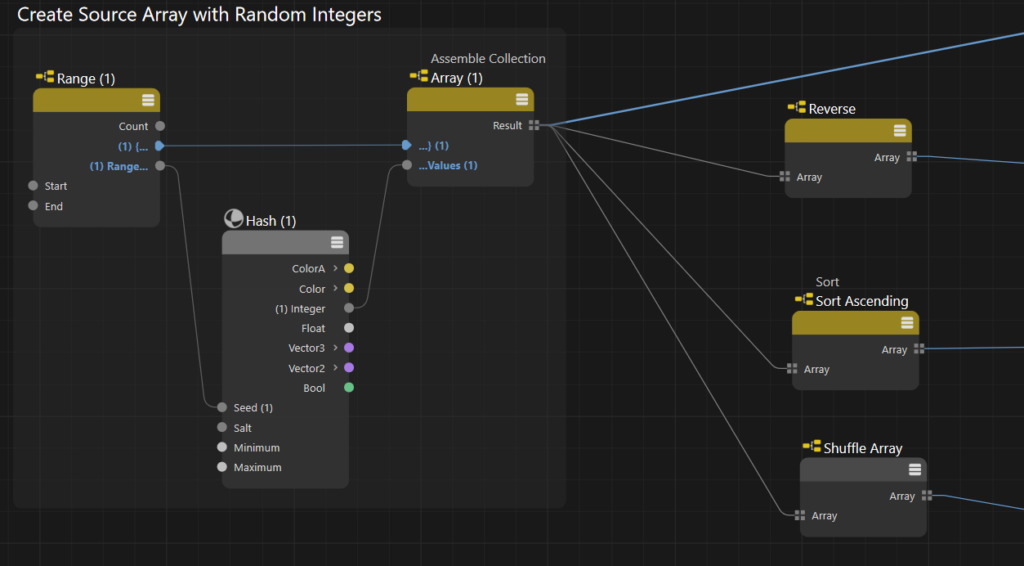
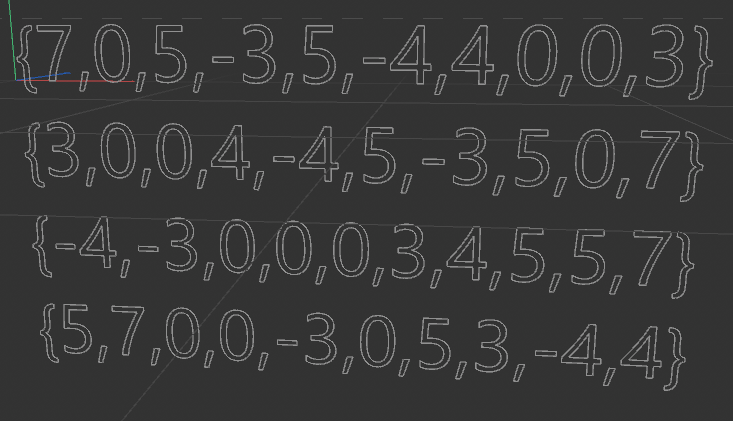
All three operations are straight forward and have no parameters beside the obvious.
Example-Changing-the-Order-of-Arrays.c4d
Deleting Elements with specific Values
Erase Value will remove all elements from an array that hold a specific value
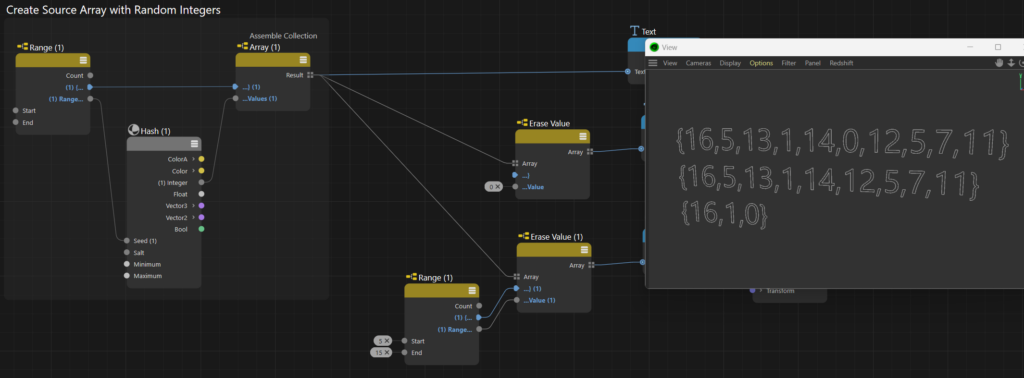
This can be either a single value or an iteration of values. The example shows the removal of all elements with the value 0, as well as the removal of all elements with values from 5-14.
Take Away
- When adding or removing elements of an array make sure that afterwards the correct count and content is taken into account
- Deleting elements can be slow, if you need to delete from the end use Truncate instead
- Deleting elements from the middle of an array is very slow, if the order of elements is not important use Swap Erase
- Use the Heat Map function to find bottlenecks