Combining Arrays
The straight forward way to combine two or more arrays is the Concatenate node. It has a Variadic input to add any number of arrays to the first one provided.
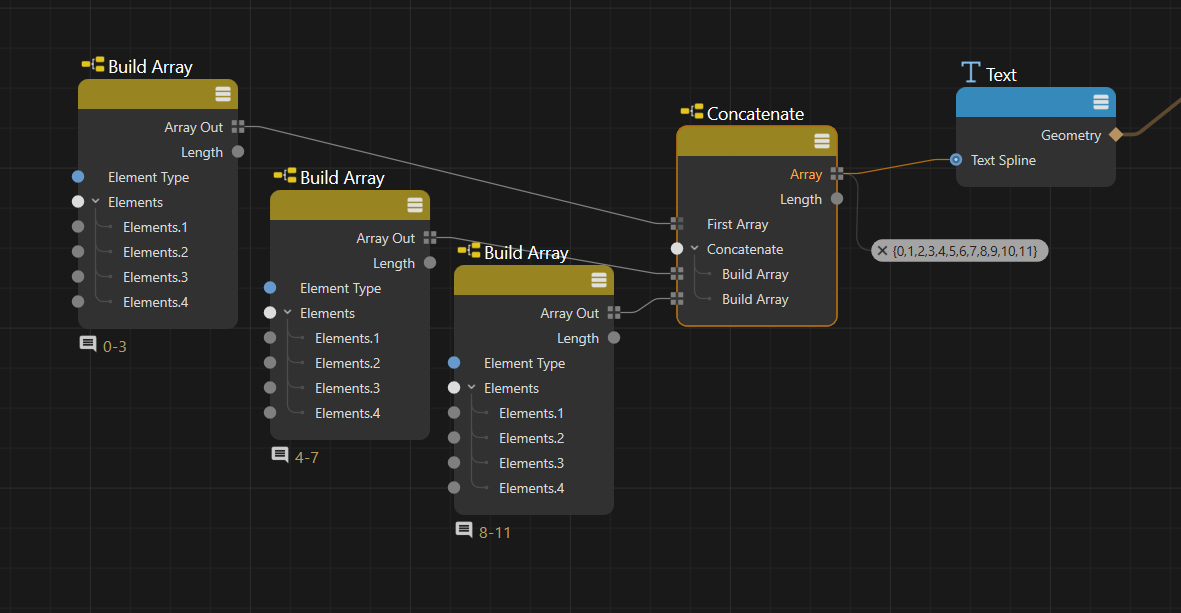
Of course this will only work if all arrays are available as is and if their number is not changing.
Things get a bit more complicated if the arrays come from an iteration or a more complex data structure like an array of arrays.
Here we have an iterator that creates a number of different arrays by adding a unique value per iteration to every element, we don’t necessarily know their final number and they come as a stream/iteration of values so a solution using a number of variadic inputs for Concatenate doesn’t work.
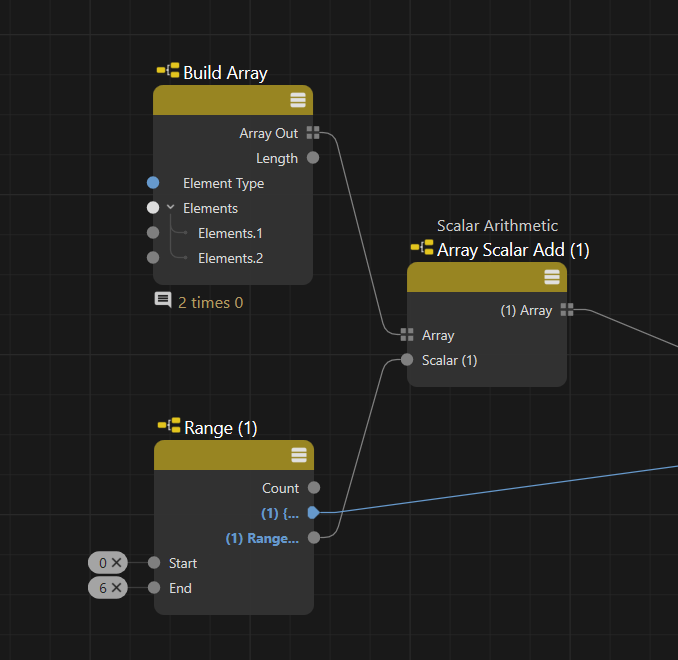
Cue the most helpful node when it comes to ending iterations and generating results from them, the Loop Carried Value node.
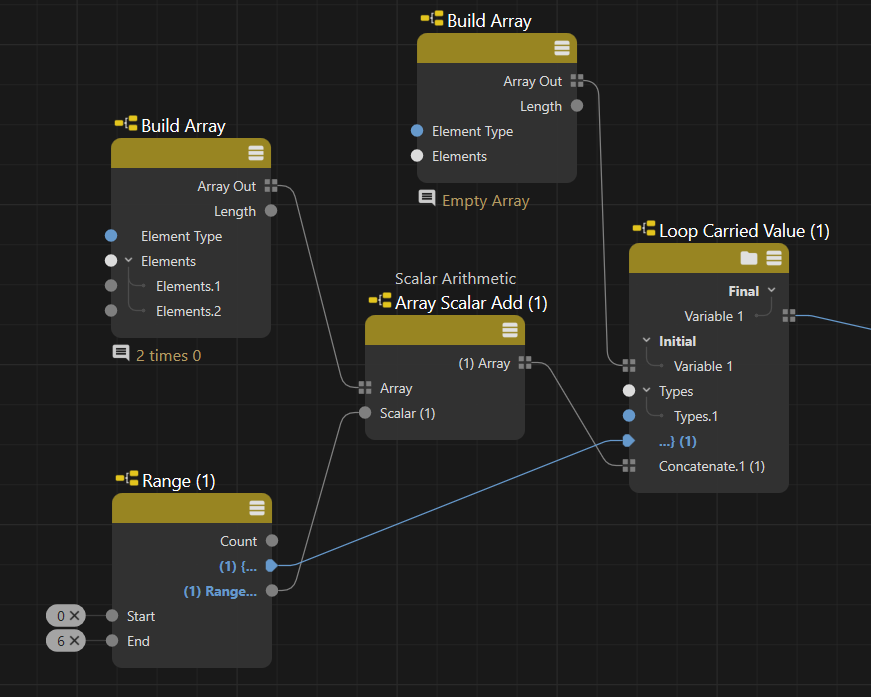
The inside of the LCV couldn’t be much simpler, the Concatenate node is used to add the iterated array at the end of the loop variable array.
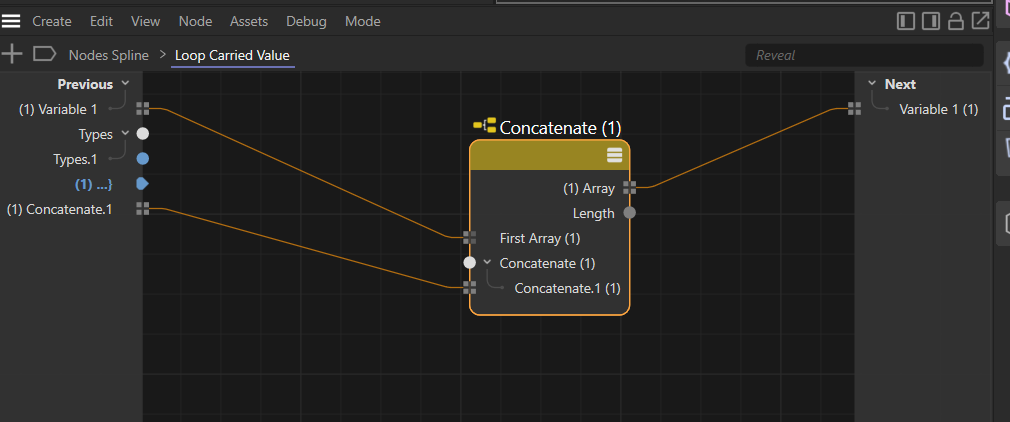
Of note here is the fact that for complex datatypes we need to provide the initial data structure for the LCV result, in this case an empty Array of Integer.
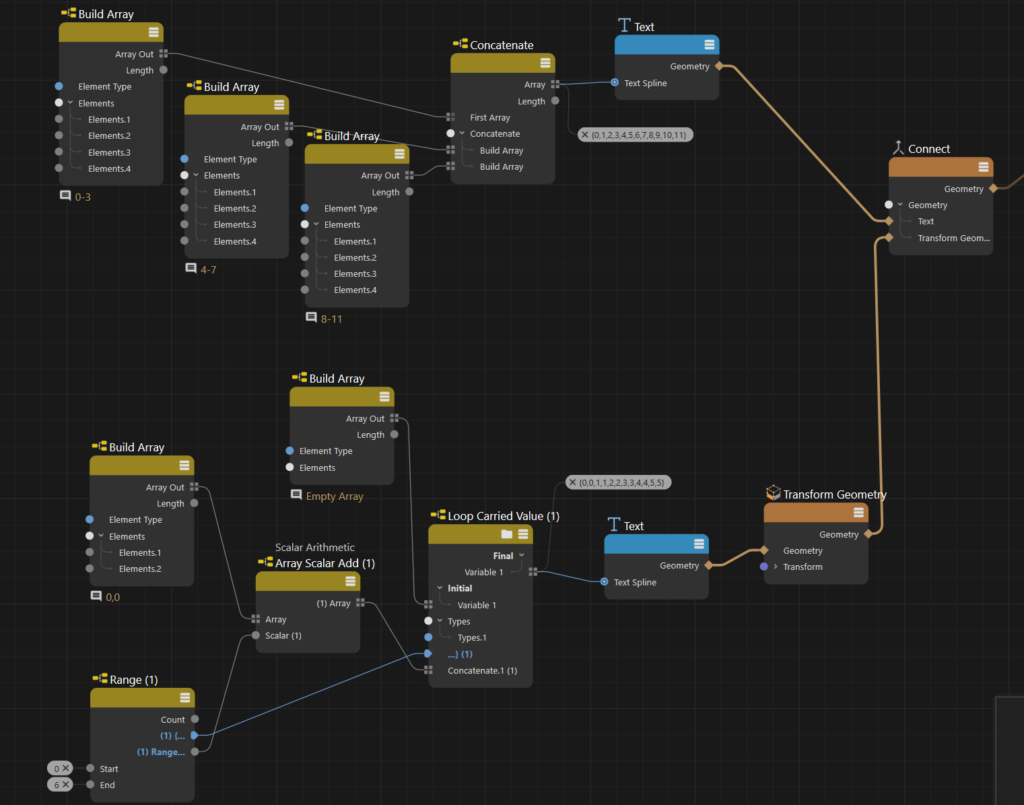
Extending Arrays
Adding elements at the end of an Array
Sometimes it is necessary to add single elements at the start or end of an array, i.e. in case of extending a Spline
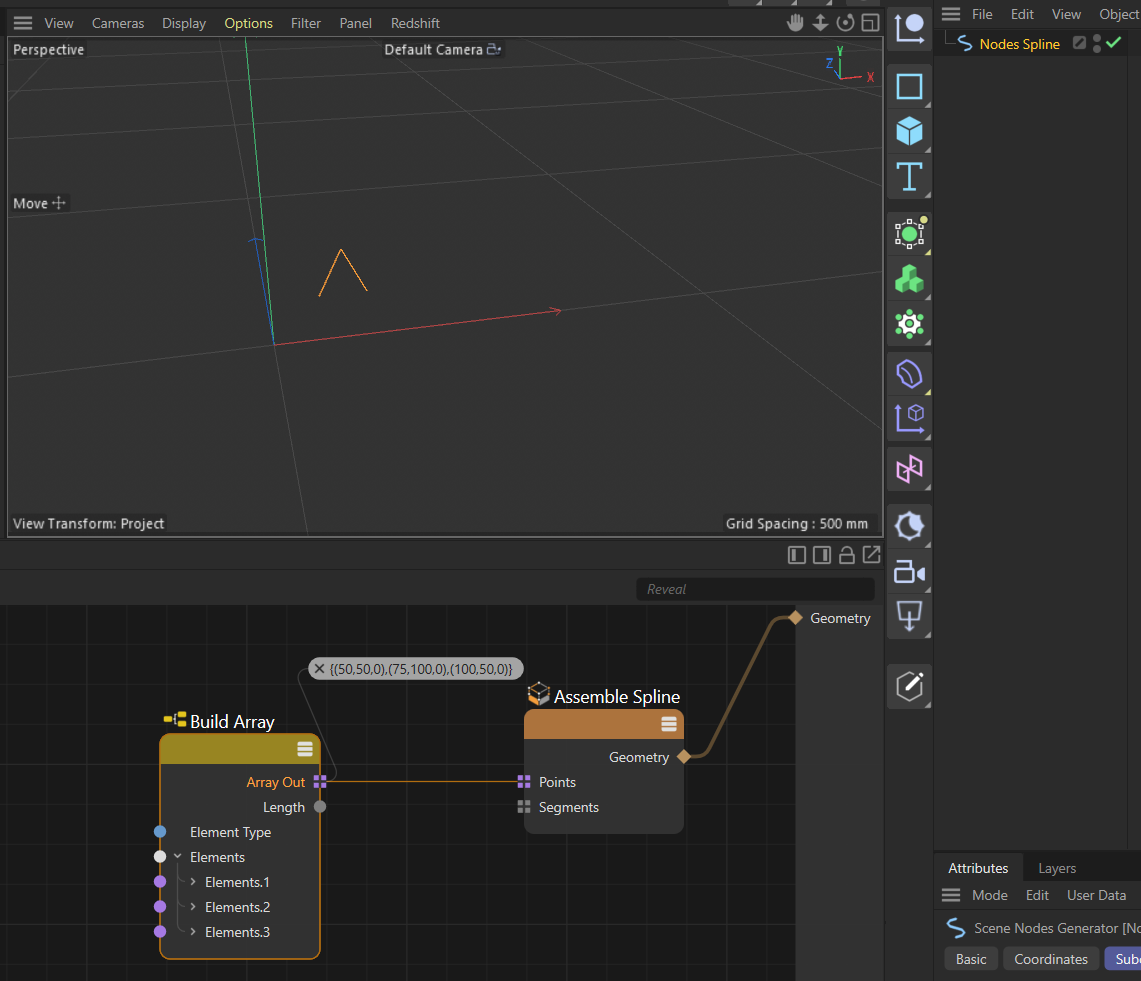
To add a single element at the end of an Array the Append Elements does a good job
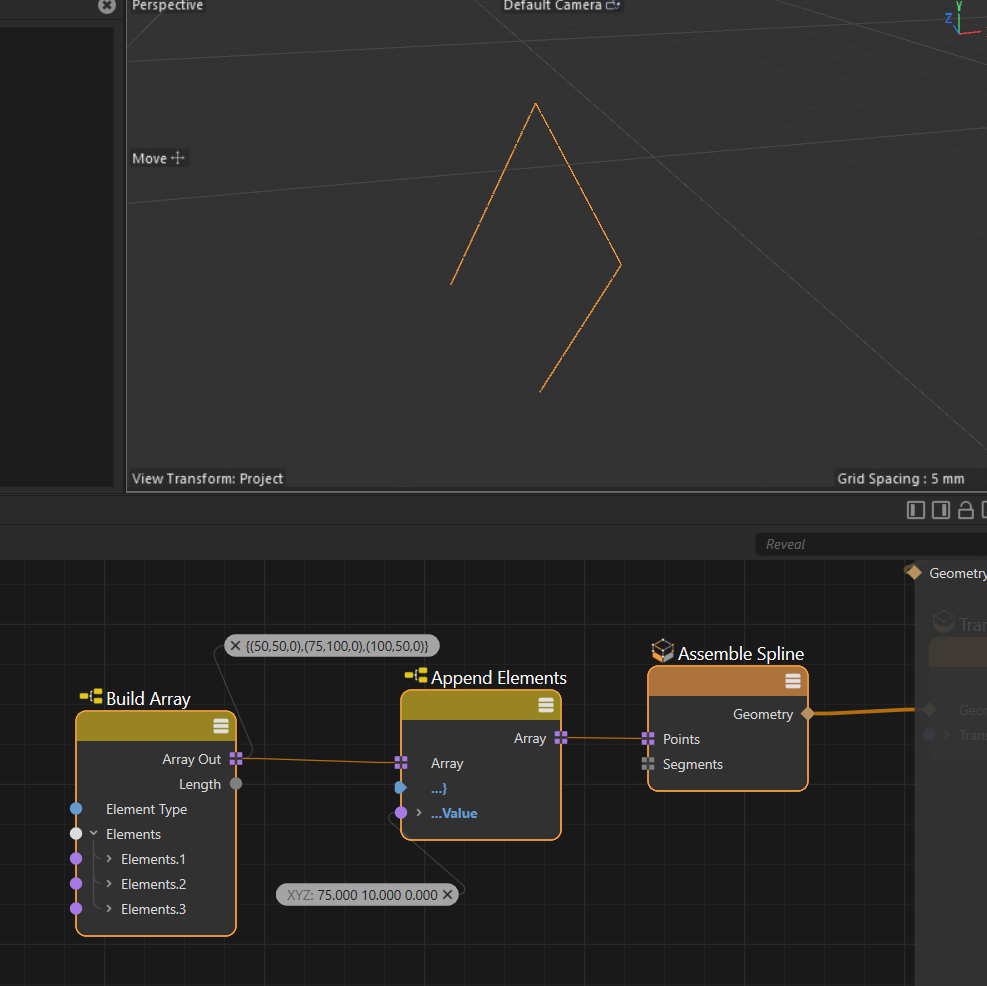
From the availability of the …} port you can see that this node can also work as the end point of an iteration. Here is how this can work.
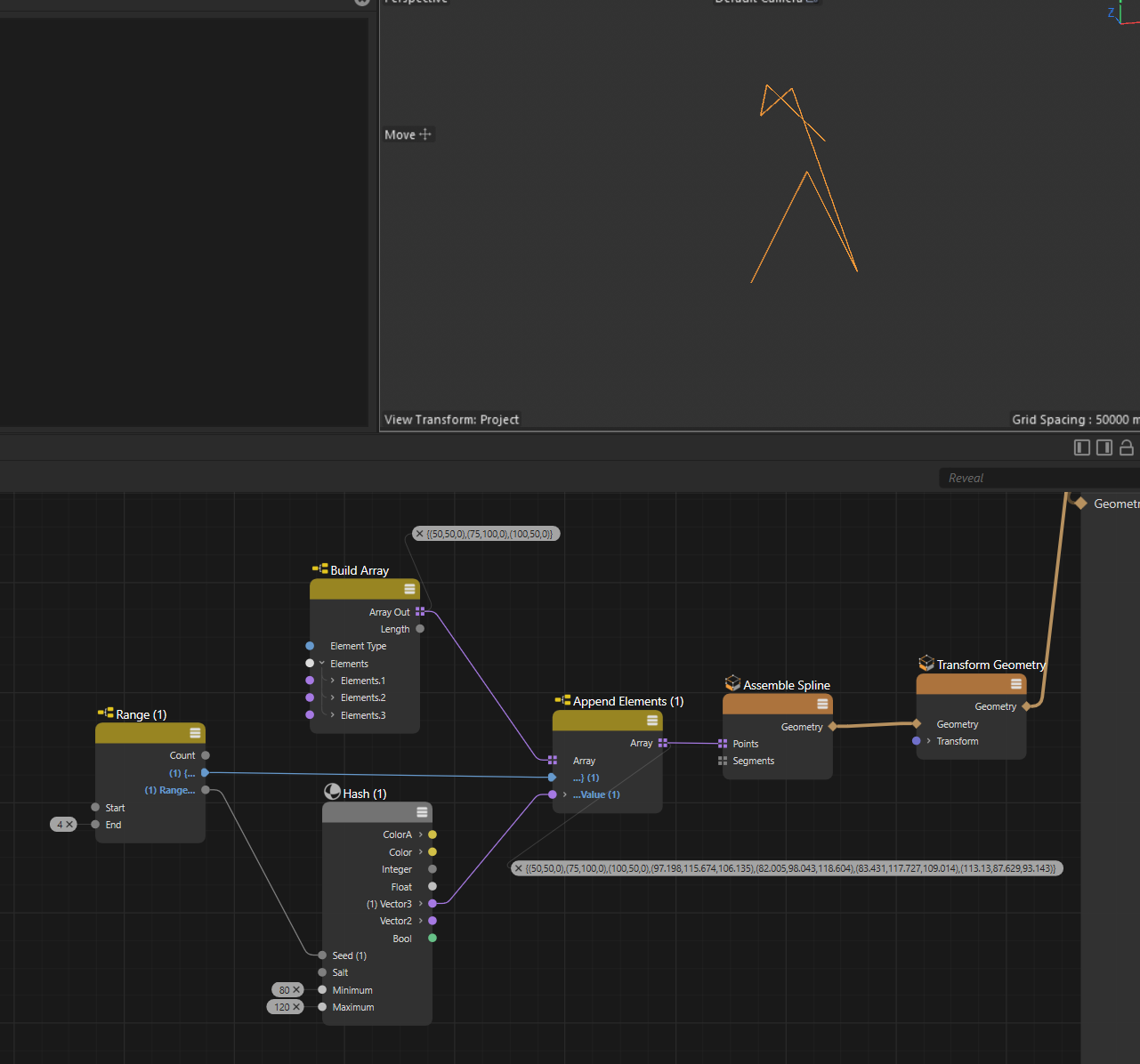
The Hash node is used to create a pseudo random vector, by controlling the Seed with the Range port we get a unique position for each iteration step. With this we add four new points to the Array and therefore the Spline.
Example-Extending-Arrays-at-End.c4d
The Append Elements node has some nice extra options, you can control the number of times the value is added. This can be used to create or add to Arrays based on a condition. An example on this can be found here
Conditional Creation of Arrays
Adding elements at the start of an array
There is no solution for this situation that is as straight forward as the Appen Element node, but it is also a situation that come sup a lot less often than adding to the end of an array.
The solution here is the Concatenate node in combination with a node that turns the value(s) we want to add into an array.
Prime choice is the Build Array node. It offers a variadic input to add any number of elements.
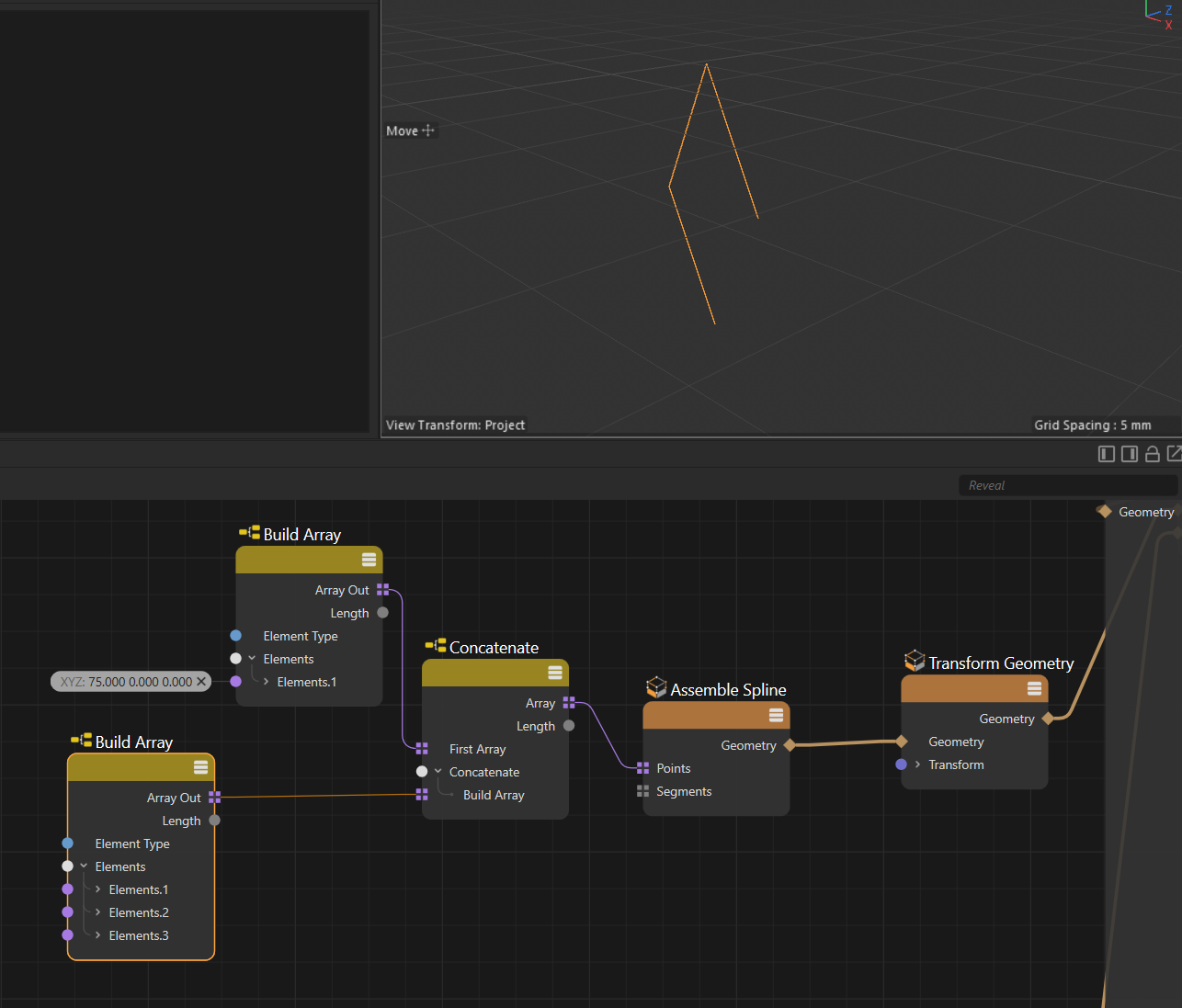
Example-Extending-Arrays-at-Beginning.c4d
It is easy to see that any number of elements can be added by extending the variadic ports.
An Iterative solution would combine the iterated values to an own array, which is then combined with the first one using Concatenate.
Assembling Arrays from Iterations
In the above examples we have assembled Arrays from iterations using the Append Elements node. This is a valid approach and actually the preferred one if you need to append conditionally.
If however all elements of an iteration need to be combined to an array there is a better way to do this, the Assemble Collection node.
Assemble Collection can do more than assemble arrays, collections aren’t necessarily Arrays, they can be a lot more varied.
Different to the Build Array node we need to provide the complete datatype here, this means choosing Array as a structure for the datatype we want to collect in the array
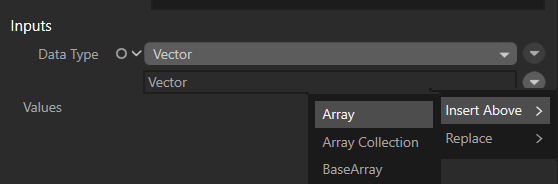
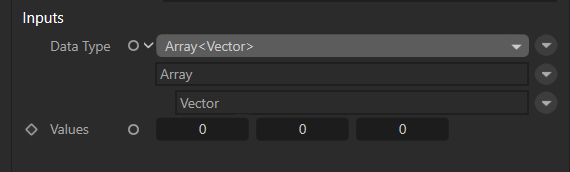
On a side note, expert users can also provide the datatype as a string, the drop down for the Data Type offers an Edit option. This would be the string needed to create the above array
net.maxon.parametrictype.vec<3,float>[]C
With the Assemble Collection node prepared we can now use it to create an Array from an iteration of vectors, for creation of the positions the same setup for random vectors as before is used.
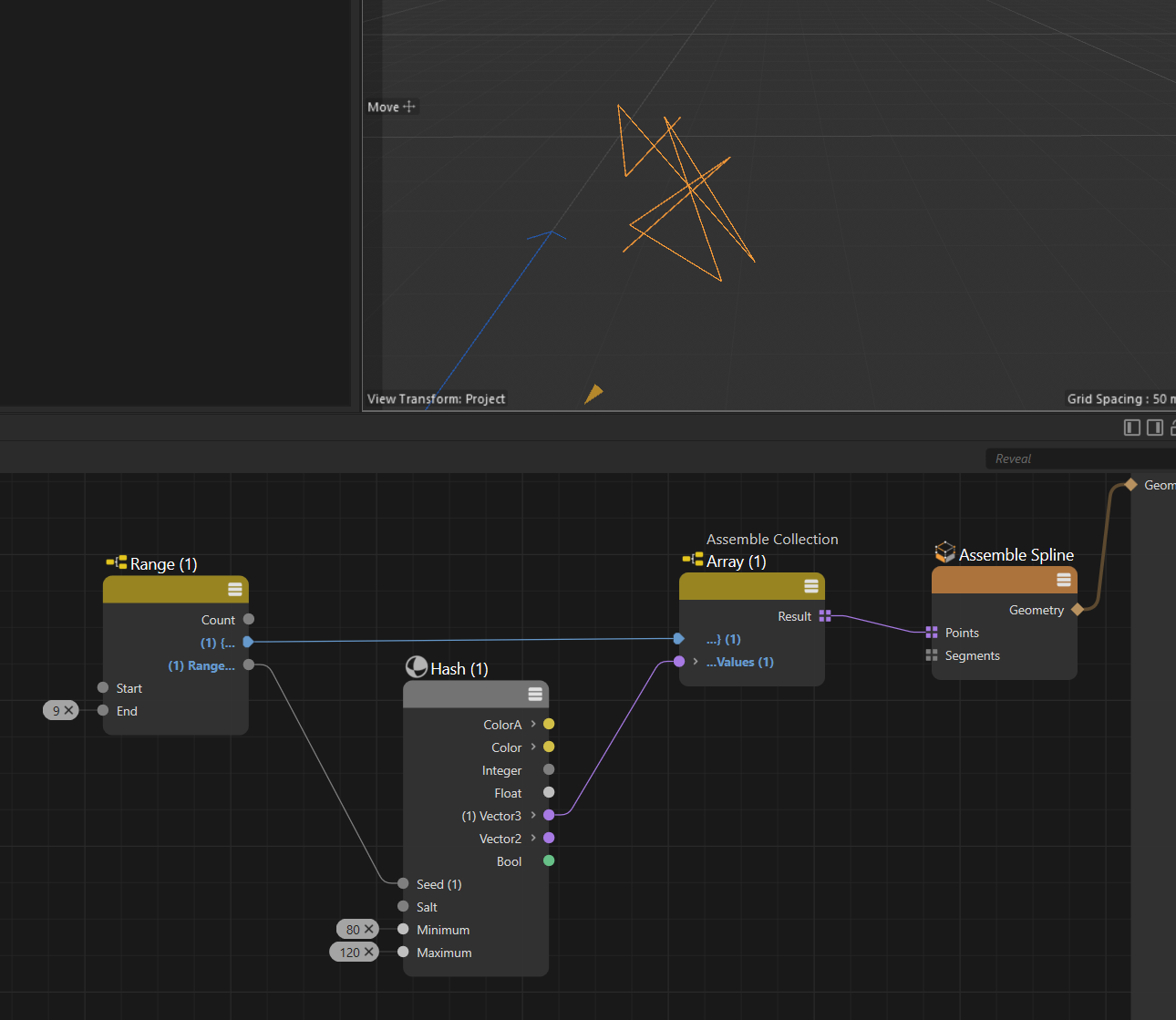
In general Assemble Collection is preferable over the Append Elements approach, since it can be better optimized by the internal system.